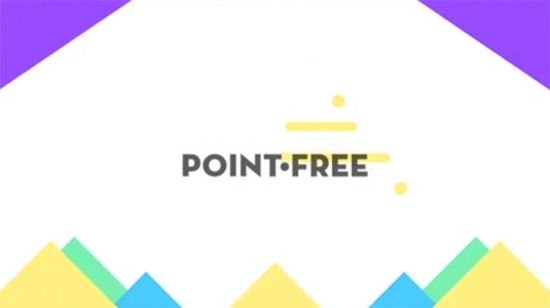
Free Download Point-Free - A video series exploring functional programming and Swift (Updated 03.2025)
Brandon Williams, Stephen Celis | Duration: ~37'x319 | Video: H264 1920x1080 | Audio: AAC 48 kHz 2ch | 115,9 GB | Language: English
Point-Free is here, bringing you videos covering functional programming concepts using the Swift language.
Point-Free is a video series covering functional programming and Swift. We've been working in functional programming for quite some time now, and we've seen a lot of benefits. So, we've wanted to share with more people, and what better way than a video series that we can bring to our community.
Functional programming leads to a lot of interesting ways to reuse code and make code more testable. But, we didn't even know about many of these ideas for most of our careers. However, by diving deeper and deeper things started to slowly make more sense it became clear that this was a serious tool for wiping away complexity that every programming should have at their disposal.
Unfortunately, functional programming is sometimes seen as overly academic or unapproachable. That's a shame because it's a really beautiful way of doing programming, just a little different from what we are used to. It emphasizes immutable values, which means you are not allowed to mutate! And it is weirdly obsessed with functions and how they compose, and in some sense that is all that matters.
Contents
000. We launched!
001. Functions
002. Side-Effects
003. UIKit Styling with Functions
004. Algebraic Data Types
005. Higher-Order Functions
006. Functional Setters
007. Setters and Key Paths
008. Getters and Key Paths
009. Algebraic Data Types: Exponents
010. A Tale of Two Flat-Maps
011. Composition without Operators
012. Tagged
013. The Many Faces of Map
014. Contravariance
015. Setters: Ergonomics & Performance
016. Dependency Injection Made Easy
017. Styling with Overture
018. Dependency Injection Made Comfortable
019. Algebraic Data Types: Generics and Recursion
020. NonEmpty
021. Playground Driven Development
022. A Tour of Point-Free
023. The Many Faces of Zip: Part 1
024. The Many Faces of Zip: Part 2
025. The Many Faces of Zip: Part 3
026. Domain Specific Languages: Part 1
027. Domain Specific Languages: Part 2
028. An HTML DSL
029. DSLs vs. Templating Languages
030. Composable Randomness
031. Decodable Randomness: Part 1
032. Decodable Randomness: Part 2
033. Protocol Witnesses: Part 1
034. Protocol Witnesses: Part 2
035. Advanced Protocol Witnesses: Part 1
036. Advanced Protocol Witnesses: Part 2
037. Protocol-Oriented Library Design: Part 1
038. Protocol-Oriented Library Design: Part 2
039. Witness-Oriented Library Design
040. Async Functional Refactoring
041. A Tour of Snapshot Testing
042. The Many Faces of Flat-Map: Part 1
043. The Many Faces of Flat-Map: Part 2
044. The Many Faces of Flat-Map: Part 3
045. The Many Faces of Flat-Map: Part 4
046. The Many Faces of Flat-Map: Part 5
047. Predictable Randomness: Part 1
048. Predictable Randomness: Part 2
049. Generative Art: Part 1
050. Generative Art: Part 2
051. Structs ? Enums
052. Enum Properties
053. Swift Syntax Enum Properties
054. Advanced Swift Syntax Enum Properties
055. Swift Syntax Command Line Tool
056. What Is a Parser?: Part 1
057. What Is a Parser?: Part 2
058. What Is a Parser?: Part 3
059. Composable Parsing: Map
060. Composable Parsing: Flat-Map
061. Composable Parsing: Zip
062. Parser Combinators: Part 1
063. Parser Combinators: Part 2
064. Parser Combinators: Part 3
065. SwiftUI and State Management: Part 1
066. SwiftUI and State Management: Part 2
067. SwiftUI and State Management: Part 3
068. Composable State Management: Reducers
069. Composable State Management: State Pullbacks
070. Composable State Management: Action Pullbacks
071. Composable State Management: Higher-Order Reducers
072. Modular State Management: Reducers
073. Modular State Management: View State
074. Modular State Management: View Actions
075. Modular State Management: The Point
076. Effectful State Management: Synchronous Effects
077. Effectful State Management: Unidirectional Effects
078. Effectful State Management: Asynchronous Effects
079. Effectful State Management: The Point
080. The Combine Framework and Effects: Part 1
081. The Combine Framework and Effects: Part 2
082. Testable State Management: Reducers
083. Testable State Management: Effects
084. Testable State Management: Ergonomics
085. Testable State Management: The Point
086. SwiftUI Snapshot Testing
087. The Case for Case Paths: Introduction
088. The Case for Case Paths: Properties
089. Case Paths for Free
090. Composing Architecture with Case Paths
091. Dependency Injection Made Composable
092. Dependency Injection Made Modular
093. Modular Dependency Injection: The Point
094. Adaptive State Management: Performance
095. Adaptive State Management: State
096. Adaptive State Management: Actions
097. Adaptive State Management: The Point
098. Ergonomic State Management: Part 1
099. Ergonomic State Management: Part 2
100. A Tour of the Composable Architecture: Part 1
101. A Tour of the Composable Architecture: Part 2
102. A Tour of the Composable Architecture: Part 3
103. A Tour of the Composable Architecture: Part 4
104. Combine Schedulers: Testing Time
105. Combine Schedulers: Controlling Time
106. Combine Schedulers: Erasing Time
107. Composable SwiftUI Bindings: The Problem
108. Composable SwiftUI Bindings: Case Paths
109. Composable SwiftUI Bindings: The Point
110. Designing Dependencies: The Problem
111. Designing Dependencies: Modularization
112. Designing Dependencies: Reachability
113. Designing Dependencies: Core Location
114. Designing Dependencies: The Point
115. SwiftUI: The Problem
116. Redacted SwiftUI: The Composable Architecture
117. The Point of Redacted SwiftUI: Part 1
118. The Point of Redacted SwiftUI: Part 2
119. Parser Combinators Recap: Part 1
120. Parser Combinators Recap: Part 2
121. Parsing Xcode Logs: Part 1
122. Parsing Xcode Logs: Part 2
123. Fluently Zipping Parsers
124. Generalized Parsing: Part 1
125. Generalized Parsing: Part 2
126. Generalized Parsing: Part 3
127. Parsing Performance: Strings
128. Parsing Performance: Combinators
129. Parsing Performance: Protocols
130. Parsing Performance: The Point
131. Concise Forms: SwiftUI
132. Concise Forms: Composable Architecture
133. Concise Forms: Bye Bye Boilerplate
134. Concise Forms: The Point
135. SwiftUI Animation: The Basics
136. SwiftUI Animation: Composable Architecture
137. SwiftUI Animation: The Point
138. Better Test Dependencies: Exhaustivity
139. Better Test Dependencies: Failability
140. Better Test Dependencies: Immediacy
141. Better Test Dependencies: The Point
142. A Tour of isowords: Part 1
143. A Tour of isowords: Part 2
144. A Tour of isowords: Part 3
145. A Tour of isowords: Part 4
146. Derived Behavior: The Problem
147. Derived Behavior: Composable-Architecture
148. Derived Behavior: Collections
149. Derived Behavior: Optionals and Enums
150. Derived Behavior: The Point
151. Composable Architecture Performance: View Stores and Scoping
152. Composable Architecture Performance: Case Paths
153. Async Refreshable: SwiftUI
154. Async Refreshable: Composable Architecture
155. SwiftUI Focus State
156. SwiftUI Searchable: Part 1
157. SwiftUI Searchable: Part 2
158. Safer, Conciser Forms: Part 1
159. Safer, Conciser Forms: Part 2
160. SwiftUI Navigation: Tabs & Alerts, Part 1
161. SwiftUI Navigation: Tabs & Alerts, Part 2
162. SwiftUI Navigation: Sheets & Popovers, Part 1
163. SwiftUI Navigation: Sheets & Popovers, Part 2
164. SwiftUI Navigation: Sheets & Popovers, Part 3
165. SwiftUI Navigation: Links, Part 1
166. SwiftUI Navigation: Links, Part 2
167. SwiftUI Navigation: Links, Part 3
168. SwiftUI Navigation: The Point
169. UIKit Navigation: Part 1
170. UIKit Navigation: Part 2
171. Modularization: Part 1
172. Modularization: Part 2
173. Parser Builders: The Problem
174. Parser Builders: The Solution
175. Parser Builders: The Point
176. Parser Errors: from Nil to Throws
177. Parser Errors: Context and Ergonomics
178. Parser Printers: The Problem
179. Parser Printers: The Solution, Part 1
180. Parser Printers: The Solution, Part 2
181. Parser Printers: Generalization
182. Parser Printers: Map
183. Parser Printers: Bizarro Printing
184. Parser Printers: The Point
185. Tour of Parser-Printers: Introduction
186. Tour of Parser-Printers: vs. Swift's Regex DSL
187. Tour of Parser-Printers: URL Routing
188. Tour of Parser-Printers: Vapor Routing
189. Tour of Parser-Printers: API Clients for Free
190. Concurrency's Past: Threads
191. Concurrency's Present: Queues and Combine
192. Concurrency's Future: Tasks and Cooperation
193. Concurrency's Future: Sendable and Actors
194. Concurrency's Future: Structured and Unstructured
195. Async Composable Architecture: The Problem
196. Async Composable Architecture: Tasks
197. Async Composable Architecture: Schedulers
198. Async Composable Architecture: Streams
199. Async Composable Architecture: Effect Lifetimes
200. Async Composable Architecture in Practice
201. Reducer Protocol: The Problem
202. Reducer Protocol: The Solution
203. Reducer Protocol: Composition, Part 1
204. Reducer Protocol: Composition, Part 2
205. Reducer Protocol: Dependencies, Part 1
206. Reducer Protocol: Dependencies, Part 2
207. Reducer Protocol: Testing
208. Reducer Protocol in Practice
209. Clocks: Existential Time
210. Clocks: Controlling Time
211. SwiftUI Navigation: Recap
212. SwiftUI Navigation: Decoupling
213. SwiftUI Navigation: Stacks
214. Modern SwiftUI: Introduction
215. Modern SwiftUI: Navigation, Part 1
216. Modern SwiftUI: Navigation, Part 2
217. Modern SwiftUI: Effects, Part 1
218. Modern SwiftUI: Effects, Part 2
219. Modern SwiftUI: Dependencies & Testing, Part 1
220. Modern SwiftUI: Dependencies & Testing, Part 2
221. Point-Free Live: Dependencies & Stacks
222. Composable Navigation: Tabs
223. Composable Navigation: Alerts & Dialogs
224. Composable Navigation: Sheets
225. Composable Navigation: Effect Cancellation
226. Composable Navigation: Unification
227. Composable Navigation: Links
228. Composable Navigation: Destinations
229. Composable Navigation: Correctness
230. Composable Navigation: Stack vs Heap
231. Composable Stacks: vs Trees
232. Composable Stacks: Multiple Layers
233. Composable Stacks: Multiple Destinations
234. Composable Stacks: Action Ergonomics
235. Composable Stacks: State Ergonomics
236. Composable Stacks: Effect Cancellation
237. Composable Stacks: Testing
238. Reliable Async Tests: The Problem
239. Reliable Async Tests: More Problems
240. Reliable Async Tests: ?
241. Reliable Async Tests: ?
242. Reliable Async Tests: The Point
243. Tour of the Composable Architecture: The Basics
244. Tour of the Composable Architecture: Introducing Standups
245. Tour of the Composable Architecture: Navigation
246. Tour of the Composable Architecture: Stacks
247. Tour of the Composable Architecture: Correctness
248. Tour of the Composable Architecture: Dependencies
249. Tour of the Composable Architecture: Persistence
250. Testing & Debugging Macros: Part 1
251. Testing & Debugging Macros: Part 2
252. Observation: The Past
253. Observation: The Present
254. Observation: The Gotchas
255. Observation: The Future
256. Observation in Practice
257. Macro Case Paths: Part 1
258. Macro Case Paths: Part 2
259. Observable Architecture: Sneak Peek
260. Observable Architecture: Structural Identity
261. Observable Architecture: Observing Optionals
262. Observable Architecture: Observing Enums
263. Observable Architecture: Observing Collections
264. Observable Architecture: Observing Navigation
265. Observable Architecture: Observing Bindings
266. Observable Architecture: The Point
267. Point-Free Live: Observation in Practice
268. Shared State: The Problem
269. Shared State: The Solution, Part 1
270. Shared State: The Solution, Part 2
271. Shared State: Testing, Part 1
272. Shared State: Testing, Part 2
273. Shared State: User Defaults, Part 1
274. Shared State: User Defaults, Part 2
275. Shared State: File Storage, Part 1
276. Shared State: File Storage, Part 2
277. Shared State in Practice: SyncUps, Part 1
278. Shared State in Practice: SyncUps: Part 2
279. Shared State in Practice: isowords, Part 1
280. Shared State in Practice: isowords, Part 2
281. Modern UIKit: Sneak Peek, Part 1
282. Modern UIKit: Sneak Peek, Part 2
283. Modern UIKit: Observation
284. Modern UIKit: Basics of Navigation
285. Modern UIKit: Unified Navigation
286. Modern UIKit: Tree-based Navigation
287. Modern UIKit: Stack Navigation, Part 1
288. Modern UIKit: Stack Navigation, Part 2
289. Modern UIKit: UIControl Bindings
290. Cross-Platform Swift: View Paradigms
291. Cross-Platform Swift: WebAssembly
292. Cross-Platform Swift: Networking
293. Cross-Platform Swift: Navigation
294. Cross-Platform Swift: UI Controls
295. Cross-Platform Swift: New Features
296. Cross-Platform Swift: Persistence
297. Back to Basics: Equatable
298. Back to Basics: Hashable
299. Back to Basics: Hashable References
300. Back to Basics: Advanced Hashable
301. SQLite: The C Library
302. SQLite: GRDB
303. SQLite: SwiftUI
304. SQLite: Observation
305. Tour of Sharing: App Storage, Part 1
306. Tour of Sharing: App Storage, Part 2
307. Tour of Sharing: File Storage: Part 1
308. Tour of Sharing: File Storage: Part 2
309. Sharing with SQLite: The Problems
310. Sharing with SQLite: The Solution
311. Sharing with SQLite: Advanced Queries
312. Sharing with SQLite: Dynamic Queries
313. Point-Free Live: SharingGRDB
314. SQL Builders: Sneak Peek, Part 1
315. SQL Builders: Sneak Peek, Part 2
316. SQL Builders: Selects
317. SQL Builders: Advanced Selects
318. SQL Builders: Order
Homepage:
Code:
https://www.pointfree.co/
Recommend Download Link Hight Speed | Please Say Thanks Keep Topic Live
AusFile
xjywh.Lessons.1-284.part01.rar.html
xjywh.Lessons.1-284.part02.rar.html
xjywh.Lessons.1-284.part03.rar.html
xjywh.Lessons.1-284.part04.rar.html
xjywh.Lessons.1-284.part05.rar.html
xjywh.Lessons.1-284.part06.rar.html
xjywh.Lessons.1-284.part07.rar.html
xjywh.Lessons.1-284.part08.rar.html
xjywh.Lessons.1-284.part09.rar.html
xjywh.Lessons.1-284.part10.rar.html
xjywh.Lessons.1-284.part11.rar.html
xjywh.Lessons.1-284.part12.rar.html
xjywh.Lessons.1-284.part13.rar.html
xjywh.Lessons.1-284.part14.rar.html
xjywh.Lessons.1-284.part15.rar.html
xjywh.Lessons.1-284.part16.rar.html
xjywh.Lessons.1-284.part17.rar.html
xjywh.Lessons.1-284.part18.rar.html
xjywh.Lessons.1-284.part19.rar.html
xjywh.Lessons.1-284.part20.rar.html
xjywh.Lessons.1-284.part21.rar.html
xjywh.Lessons.1-284.part22.rar.html
xjywh.Lessons.1-284.part23.rar.html
xjywh.Lessons.1-284.part24.rar.html
xjywh.Lessons.1-284.part25.rar.html
xjywh.Lessons.1-284.part26.rar.html
xjywh.Lessons.1-284.part27.rar.html
xjywh.Lessons.1-284.part28.rar.html
xjywh.Lessons.1-284.part29.rar.html
xjywh.Lessons.1-284.part30.rar.html
xjywh.Lessons.1-284.part31.rar.html
xjywh.Lessons.1-284.part32.rar.html
xjywh.Lessons.1-284.part33.rar.html
xjywh.Lessons.1-284.part34.rar.html
xjywh.Lessons.1-284.part35.rar.html
xjywh.Lessons.1-284.part36.rar.html
xjywh.Lessons.1-284.part37.rar.html
xjywh.Lessons.1-284.part38.rar.html
xjywh.Lessons.1-284.part39.rar.html
xjywh.Lessons.1-284.part40.rar.html
xjywh.Lessons.1-284.part41.rar.html
xjywh.Lessons.1-284.part42.rar.html
xjywh.Lessons.1-284.part43.rar.html
xjywh.Lessons.1-284.part44.rar.html
xjywh.Lessons.1-284.part45.rar.html
xjywh.Lessons.1-284.part46.rar.html
xjywh.Lessons.1-284.part47.rar.html
xjywh.Lessons.1-284.part48.rar.html
xjywh.Lessons.1-284.part49.rar.html
xjywh.Lessons.1-284.part50.rar.html
xjywh.Lessons.1-284.part51.rar.html
xjywh.Lessons.1-284.part52.rar.html
xjywh.Lessons.1-284.part53.rar.html
xjywh.UPDATE.lessons.285-318.part1.rar.html
xjywh.UPDATE.lessons.285-318.part2.rar.html
xjywh.UPDATE.lessons.285-318.part3.rar.html
xjywh.UPDATE.lessons.285-318.part4.rar.html
xjywh.UPDATE.lessons.285-318.part5.rar.html
xjywh.UPDATE.lessons.285-318.part6.rar.html
xjywh.UPDATE.lessons.285-318.part7.rar.html
Fileaxa
xjywh.Lessons.1-284.part01.rar
xjywh.Lessons.1-284.part02.rar
xjywh.Lessons.1-284.part03.rar
xjywh.Lessons.1-284.part04.rar
xjywh.Lessons.1-284.part05.rar
xjywh.Lessons.1-284.part06.rar
xjywh.Lessons.1-284.part07.rar
xjywh.Lessons.1-284.part08.rar
xjywh.Lessons.1-284.part09.rar
xjywh.Lessons.1-284.part10.rar
xjywh.Lessons.1-284.part11.rar
xjywh.Lessons.1-284.part12.rar
xjywh.Lessons.1-284.part13.rar
xjywh.Lessons.1-284.part14.rar
xjywh.Lessons.1-284.part15.rar
xjywh.Lessons.1-284.part16.rar
xjywh.Lessons.1-284.part17.rar
xjywh.Lessons.1-284.part18.rar
xjywh.Lessons.1-284.part19.rar
xjywh.Lessons.1-284.part20.rar
xjywh.Lessons.1-284.part21.rar
xjywh.Lessons.1-284.part22.rar
xjywh.Lessons.1-284.part23.rar
xjywh.Lessons.1-284.part24.rar
xjywh.Lessons.1-284.part25.rar
xjywh.Lessons.1-284.part26.rar
xjywh.Lessons.1-284.part27.rar
xjywh.Lessons.1-284.part28.rar
xjywh.Lessons.1-284.part29.rar
xjywh.Lessons.1-284.part30.rar
xjywh.Lessons.1-284.part31.rar
xjywh.Lessons.1-284.part32.rar
xjywh.Lessons.1-284.part33.rar
xjywh.Lessons.1-284.part34.rar
xjywh.Lessons.1-284.part35.rar
xjywh.Lessons.1-284.part36.rar
xjywh.Lessons.1-284.part37.rar
xjywh.Lessons.1-284.part38.rar
xjywh.Lessons.1-284.part39.rar
xjywh.Lessons.1-284.part40.rar
xjywh.Lessons.1-284.part41.rar
xjywh.Lessons.1-284.part42.rar
xjywh.Lessons.1-284.part43.rar
xjywh.Lessons.1-284.part44.rar
xjywh.Lessons.1-284.part45.rar
xjywh.Lessons.1-284.part46.rar
xjywh.Lessons.1-284.part47.rar
xjywh.Lessons.1-284.part48.rar
xjywh.Lessons.1-284.part49.rar
xjywh.Lessons.1-284.part50.rar
xjywh.Lessons.1-284.part51.rar
xjywh.Lessons.1-284.part52.rar
xjywh.Lessons.1-284.part53.rar
xjywh.UPDATE.lessons.285-318.part1.rar
xjywh.UPDATE.lessons.285-318.part2.rar
xjywh.UPDATE.lessons.285-318.part3.rar
xjywh.UPDATE.lessons.285-318.part4.rar
xjywh.UPDATE.lessons.285-318.part5.rar
xjywh.UPDATE.lessons.285-318.part6.rar
xjywh.UPDATE.lessons.285-318.part7.rar
TakeFile
xjywh.Lessons.1-284.part01.rar.html
xjywh.Lessons.1-284.part02.rar.html
xjywh.Lessons.1-284.part03.rar.html
xjywh.Lessons.1-284.part04.rar.html
xjywh.Lessons.1-284.part05.rar.html
xjywh.Lessons.1-284.part06.rar.html
xjywh.Lessons.1-284.part07.rar.html
xjywh.Lessons.1-284.part08.rar.html
xjywh.Lessons.1-284.part09.rar.html
xjywh.Lessons.1-284.part10.rar.html
xjywh.Lessons.1-284.part11.rar.html
xjywh.Lessons.1-284.part12.rar.html
xjywh.Lessons.1-284.part13.rar.html
xjywh.Lessons.1-284.part14.rar.html
xjywh.Lessons.1-284.part15.rar.html
xjywh.Lessons.1-284.part16.rar.html
xjywh.Lessons.1-284.part17.rar.html
xjywh.Lessons.1-284.part18.rar.html
xjywh.Lessons.1-284.part19.rar.html
xjywh.Lessons.1-284.part20.rar.html
xjywh.Lessons.1-284.part21.rar.html
xjywh.Lessons.1-284.part22.rar.html
xjywh.Lessons.1-284.part23.rar.html
xjywh.Lessons.1-284.part24.rar.html
xjywh.Lessons.1-284.part25.rar.html
xjywh.Lessons.1-284.part26.rar.html
xjywh.Lessons.1-284.part27.rar.html
xjywh.Lessons.1-284.part28.rar.html
xjywh.Lessons.1-284.part29.rar.html
xjywh.Lessons.1-284.part30.rar.html
xjywh.Lessons.1-284.part31.rar.html
xjywh.Lessons.1-284.part32.rar.html
xjywh.Lessons.1-284.part33.rar.html
xjywh.Lessons.1-284.part34.rar.html
xjywh.Lessons.1-284.part35.rar.html
xjywh.Lessons.1-284.part36.rar.html
xjywh.Lessons.1-284.part37.rar.html
xjywh.Lessons.1-284.part38.rar.html
xjywh.Lessons.1-284.part39.rar.html
xjywh.Lessons.1-284.part40.rar.html
xjywh.Lessons.1-284.part41.rar.html
xjywh.Lessons.1-284.part42.rar.html
xjywh.Lessons.1-284.part43.rar.html
xjywh.Lessons.1-284.part44.rar.html
xjywh.Lessons.1-284.part45.rar.html
xjywh.Lessons.1-284.part46.rar.html
xjywh.Lessons.1-284.part47.rar.html
xjywh.Lessons.1-284.part48.rar.html
xjywh.Lessons.1-284.part49.rar.html
xjywh.Lessons.1-284.part50.rar.html
xjywh.Lessons.1-284.part51.rar.html
xjywh.Lessons.1-284.part52.rar.html
xjywh.Lessons.1-284.part53.rar.html
xjywh.UPDATE.lessons.285-318.part1.rar.html
xjywh.UPDATE.lessons.285-318.part2.rar.html
xjywh.UPDATE.lessons.285-318.part3.rar.html
xjywh.UPDATE.lessons.285-318.part4.rar.html
xjywh.UPDATE.lessons.285-318.part5.rar.html
xjywh.UPDATE.lessons.285-318.part6.rar.html
xjywh.UPDATE.lessons.285-318.part7.rar.html
Rapidgator
PointFreeAvideoseriesexploringfunctionalprogrammingandSwift.html
http://peeplink.in/ea38cc15585b
Fikper
xjywh.Lessons.1-284.part01.rar.html
xjywh.Lessons.1-284.part02.rar.html
xjywh.Lessons.1-284.part03.rar.html
xjywh.Lessons.1-284.part04.rar.html
xjywh.Lessons.1-284.part05.rar.html
xjywh.Lessons.1-284.part06.rar.html
xjywh.Lessons.1-284.part07.rar.html
xjywh.Lessons.1-284.part08.rar.html
xjywh.Lessons.1-284.part09.rar.html
xjywh.Lessons.1-284.part10.rar.html
xjywh.Lessons.1-284.part11.rar.html
xjywh.Lessons.1-284.part12.rar.html
xjywh.Lessons.1-284.part13.rar.html
xjywh.Lessons.1-284.part14.rar.html
xjywh.Lessons.1-284.part15.rar.html
xjywh.Lessons.1-284.part16.rar.html
xjywh.Lessons.1-284.part17.rar.html
xjywh.Lessons.1-284.part18.rar.html
xjywh.Lessons.1-284.part19.rar.html
xjywh.Lessons.1-284.part20.rar.html
xjywh.Lessons.1-284.part21.rar.html
xjywh.Lessons.1-284.part22.rar.html
xjywh.Lessons.1-284.part23.rar.html
xjywh.Lessons.1-284.part24.rar.html
xjywh.Lessons.1-284.part25.rar.html
xjywh.Lessons.1-284.part26.rar.html
xjywh.Lessons.1-284.part27.rar.html
xjywh.Lessons.1-284.part28.rar.html
xjywh.Lessons.1-284.part29.rar.html
xjywh.Lessons.1-284.part30.rar.html
xjywh.Lessons.1-284.part31.rar.html
xjywh.Lessons.1-284.part32.rar.html
xjywh.Lessons.1-284.part33.rar.html
xjywh.Lessons.1-284.part34.rar.html
xjywh.Lessons.1-284.part35.rar.html
xjywh.Lessons.1-284.part36.rar.html
xjywh.Lessons.1-284.part37.rar.html
xjywh.Lessons.1-284.part38.rar.html
xjywh.Lessons.1-284.part39.rar.html
xjywh.Lessons.1-284.part40.rar.html
xjywh.Lessons.1-284.part41.rar.html
xjywh.Lessons.1-284.part42.rar.html
xjywh.Lessons.1-284.part43.rar.html
xjywh.Lessons.1-284.part44.rar.html
xjywh.Lessons.1-284.part45.rar.html
xjywh.Lessons.1-284.part46.rar.html
xjywh.Lessons.1-284.part47.rar.html
xjywh.Lessons.1-284.part48.rar.html
xjywh.Lessons.1-284.part49.rar.html
xjywh.Lessons.1-284.part50.rar.html
xjywh.Lessons.1-284.part51.rar.html
xjywh.Lessons.1-284.part52.rar.html
xjywh.Lessons.1-284.part53.rar.html
xjywh.UPDATE.lessons.285-318.part1.rar.html
xjywh.UPDATE.lessons.285-318.part2.rar.html
xjywh.UPDATE.lessons.285-318.part3.rar.html
xjywh.UPDATE.lessons.285-318.part4.rar.html
xjywh.UPDATE.lessons.285-318.part5.rar.html
xjywh.UPDATE.lessons.285-318.part6.rar.html
xjywh.UPDATE.lessons.285-318.part7.rar.html
No Password - Links are Interchangeable